Computer Graphics- Cheatsheet
Because I'm always forgetting this stuff
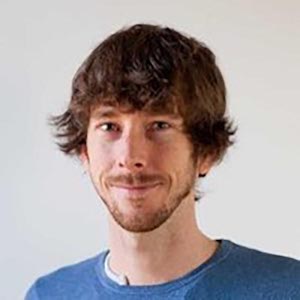
Will Perkins
Homogeneous coordinates
Adding a fourth term: \(w\) makes it easier to work in 3D euclidian space. $$({x \over w},{y \over w},{z \over w}) \Rightarrow (x,y,z,w)$$
Why? Here's a long list of reasons, but a couple key points:
- 3D scaling, rotation, and translation operations can be represented as a single linear transform (4x4 matrix)
- A direction (Infinity) can be represented in 3D space: (x,y,z,0)
Transformations
Translation
for a point \(p\): $$ T(t)p = \begin{bmatrix} 1 & 0 & 0 & t_x\\ 0 & 1 & 0 & t_y\\ 0 & 0 & 1 & t_z\\ 0 & 0 & 0 & 1\\ \end{bmatrix} \begin{bmatrix} p_x\\ p_y\\ p_z\\ 1\\ \end{bmatrix} = \begin{bmatrix} p_x + t_x\\ p_y + t_y\\ p_z + t_z\\ 1\\ \end{bmatrix} $$ for a vector \(v\): $$ T(t)v = \begin{bmatrix} 1 & 0 & 0 & t_x\\ 0 & 1 & 0 & t_y\\ 0 & 0 & 1 & t_z\\ 0 & 0 & 0 & 1\\ \end{bmatrix} \begin{bmatrix} v_x\\ v_y\\ v_z\\ 0\\ \end{bmatrix} = \begin{bmatrix} v_x\\ v_y\\ v_z\\ 0\\ \end{bmatrix} $$ inverse transform: \(T^{-1}(t) = T(-t)\)
Scaling
$$ S(t)p = \begin{bmatrix} s_x & 0 & 0 & 0\\ 0 & s_y & 0 & 0\\ 0 & 0 & s_z & 0\\ 0 & 0 & 0 & 1\\ \end{bmatrix} \begin{bmatrix} p_x\\ p_y\\ p_z\\ 1\\ \end{bmatrix} = \begin{bmatrix} s_xp_x\\ s_yp_y\\ s_zp_z\\ 1\\ \end{bmatrix} $$
Rotation
When applying individual rotations (yaw, pitch, roll), the order of rotation operations is important. To get around this problem use axis-angle representation.
If you only need to rotate around a single axis, then applying the rotation transform can be straightforward.
The inverse of a rotation matrix corresponds to its transpose
Coordinate Systems
Local space, World space, View space, Clip space, Screen space
More on Coordinate Systems
Perspective vs Orthographic Projection
perspective / orthographic projection. near plane / far plane. clipping.
Screen Space
You can use the Viewport Tranform to get to Screen Space (actual pixels)
Combining Transformations
To transform a vertex coordinate to clip coordinates: $$V_\text{clip} = M_\text{projection}\cdot{M_\text{view}}\cdot{M_\text{model}}\cdot{V_\text{local}}$$
Other Useful References
Coordinate systems we commonly reference for development.
Pinhole camera model
$$ \begin{align} P & = \overbrace{K}^{\text{Intrinsic Matrix}} \times \overbrace{\left[R|\mathbf{t}\right]}^{\text{Extrinsic Matrix}} \\ & = \overbrace{ \underbrace{ \begin{bmatrix} 1 & 0 & x_0\\ 0 & 1 & y_0\\ 0 & 0 & 1\\ \end{bmatrix} }_{\text{2D Translation}} \times \underbrace{ \begin{bmatrix} f_x & 0 & 1\\ 0 & f_y & 1\\ 0 & 0 & 1\\ \end{bmatrix} }_{\text{2D Scaling}} \times \underbrace{ \begin{bmatrix} 1 & s/f_x & 0\\ 0 & 1 & 0\\ 0 & 0 & 1\\ \end{bmatrix} }_{\text{2D Shear}} }^{\text{Intrinsic Matrix}} \times \overbrace{ \underbrace{ \begin{bmatrix} 1 & 0 & 0 & t_x\\ 0 & 1 & 0 & t_y\\ 0 & 0 & 1 & t_z\\ \end{bmatrix} }_{\text{3D Translation}} \times \underbrace{ \begin{bmatrix} ... \end{bmatrix} }_{\text{3D Rotation}} }^{\text{Extrinsic Matrix}} \end{align} $$
Source: Kyle Simek's excellent computer vision blog
FAQs
How do I get the camera direction from a given view matrix?
Column 2 of the view matrix is the camera's -Z direction. \begin{bmatrix} u_x & v_x & -n_x & -\text{eye}_x\\ u_y & v_y & -n_y & -\text{eye}_y\\ u_z & v_z & -n_z & -\text{eye}_z\\ 0 & 0 & 0 & 1\\ \end{bmatrix} Where \(u\), \(v\), and \(n\) are the normalized vectors for the camera referential. \(u\) is the up vector, \(n\) is the direction the camera is looking at, and \(v\) is perpendicular to both \(n\) and \(u\).